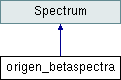
Typedefs | |
typedef std::shared_ptr< Spectrum > | SP |
typedef std::shared_ptr< const Spectrum > | SCP |
typedef std::function< SP_Vec_Dbl(const Vec_Dbl &, const Vec_Int &, const Vec_Dbl &)> | Integrator_t |
Basic Methods | |
const Vec_Dbl & | bounds () const |
return a const reference to bounds More... | |
void | set_bounds (const Vec_Dbl &) |
set the bounds (copy operation) More... | |
const Vec_Dbl & | intensity () const |
return a const reference to intensity More... | |
void | set_intensity (const Vec_Dbl &) |
set the intensity (copy operation) More... | |
Comparison | |
bool | match (const Spectrum &) const |
bool | operator== (const Spectrum &) const |
static bool | match (const Spectrum &, const Spectrum &) |
Convenience Methods | |
double | total_intensity () const |
EnergyRange_t | energy_range () const |
double | mean_energy () const |
double | total_energy_intensity () const |
SP_Vec_Dbl | newsp_energy_intensity () const |
Memory-usage Variants of Other Methods | |
Vec_Dbl * | newptr_energy_intensity () const |
SCP_Vec_Dbl | scp_intensity () const |
return a shared (read-only) intensity pointer More... | |
SCP_Vec_Dbl | scp_bounds () const |
return a shared (read-only) bounds pointer More... | |
Mapping Methods | |
bool | map_intensity (const Vec_Dbl &new_bounds, Integrator_t integrator, Vec_Dbl *intensity, double wt=1.0, double tol=ETOL) const |
bool | map_intensity (const Vec_Dbl &new_bounds, Vec_Dbl *intensity, double wt=1.0, double tol=ETOL) const |
void | remap_intensity (Vec_Dbl *intensity, double wt=1.0) const |
bool | has_mapping_variables () const |
void | clear_mapping_variables () const |
std::string | print_bins () const |
Additional Methods for Language Bindings (e.g. Fortran) | |
double | bounds_at (size_t) const |
get element of bounds vector by index More... | |
double const * | bounds_array () const |
return view of bounds vector More... | |
size_t | bounds_size () const |
return size of bounds vector More... | |
bool | has_bounds () const |
return whether the class has a non-null bounds More... | |
void | get_bounds (Vec_Dbl &) const |
populate an existing Vec_Dbl with a copy of bounds More... | |
void | manageptr_bounds (Vec_Dbl *&) |
set the bounds (ownership transfer) More... | |
void | getptr_bounds (Vec_Dbl const *&) const |
retrieve pointer (read-only) to bounds More... | |
void | get_bounds_array (size_t, double *) const |
get array of bounds vector (Fortran) More... | |
void | set_bounds_array (size_t, double *) |
set bounds vector from array (Fortran) More... | |
double | intensity_at (size_t) const |
get element of intensity vector by index More... | |
double const * | intensity_array () const |
return view of intensity vector More... | |
size_t | intensity_size () const |
return size of intensity vector More... | |
bool | has_intensity () const |
return whether the class has a non-null intensity More... | |
void | get_intensity (Vec_Dbl &) const |
populate an existing Vec_Dbl with a copy of intensity More... | |
void | manageptr_intensity (Vec_Dbl *&) |
set the intensity (ownership transfer) More... | |
void | getptr_intensity (Vec_Dbl const *&) const |
retrieve pointer (read-only) to intensity More... | |
void | get_intensity_array (size_t, double *) const |
get array of intensity vector (Fortran) More... | |
void | set_intensity_array (size_t, double *) |
set intensity vector from array (Fortran) More... | |
Static Helper Functions | |
returns true if the new and old bounds have the same min/max energy, i.e. the mapping will be conservative | |
static void | map_intensity (const Vec_Dbl &old_bounds, const Vec_Dbl &new_bounds, const Vec_Int &union_to_old, const Vec_Int &union_to_new, const Vec_Dbl &union_grid, const Vec_Dbl &old_intensity, const Vec_Dbl &union_wts, Vec_Dbl *new_intensity, double wt=1.0) |
static std::string | print_bins (const Vec_Dbl &bounds, const Vec_Dbl &intensity) |
static bool | map_bins (const Vec_Dbl &old_bounds, const Vec_Dbl &new_bounds, Vec_Int *union_to_old, Vec_Int *union_to_new, Vec_Dbl *union_grid, double tol=ETOL) |
static SP_Vec_Dbl | computeUnionWts_1 (const Vec_Dbl &union_grid, const Vec_Int &union_to_old, const Vec_Dbl &old_flux) |
Other | |
SP_Vec_Dbl | b_bounds |
SP_Vec_Dbl | b_intensity |
void | canonicalize_bounds () |
void | canonicalize_intensity () |
Public Member Functions | |
procedure, pass(this) | set_nuclide_type => Origen_BetaSpectra_set_nuclide_type |
procedure, pass(this) | nuclide_type => Origen_BetaSpectra_nuclide_type |
procedure, pass(this) | initialize => Origen_BetaSpectra_initialize |
procedure, pass(this) | destroy => Origen_BetaSpectra_destroy |
Member Typedef Documentation
|
inherited |
Member Function/Subroutine Documentation
procedure, pass(this) set_nuclide_type | ( | ) |
procedure, pass(this) nuclide_type | ( | ) |
procedure, pass(this) initialize | ( | ) |
procedure, pass(this) destroy | ( | ) |
|
inherited |
return a const reference to bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of Spectrum goes out of scope.
Referenced by Spectrum::operator==(), and StateSetIO_bof::write().
|
inherited |
set the bounds (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
- Examples:
- tstEmissionResource.cpp, tstEmissionSpectrum.cpp, and tstGammaResource.cpp.
Referenced by FakeFactory::newspStateSet_10nuclide(), StateSetIO_s62b::read(), GammaResourceIO_cimg::read(), StateSetIO_bof::read(), StateSetIO_s61::read(), FakeFactory::StateSet_random1(), and TEST().
|
inherited |
return a const reference to intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of Spectrum goes out of scope.
Referenced by Spectrum::operator==(), and StateSetIO_bof::write().
|
inherited |
set the intensity (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by FakeFactory::newspStateSet_10nuclide(), StateSetIO_s62b::read(), StateSetIO_s61::read(), StateSetIO_bof::read(), GammaResourceIO_cimg::read(), and FakeFactory::StateSet_random1().
|
inherited |
Referenced by NeutronSpectra::match(), BetaSpectra::match(), GammaSpectra::match(), and AlphaSpectra::match().
References Spectrum::b_bounds.
|
inherited |
References Spectrum::b_bounds, Spectrum::b_intensity, Spectrum::bounds(), and Spectrum::intensity().
Referenced by AlphaSpectra::operator==(), GammaSpectra::operator==(), and BetaSpectra::operator==().
|
inherited |
References Spectrum::b_intensity, and Spectrum::has_intensity().
Referenced by Spectrum::mean_energy(), and EmissionSpectrum::total_intensity().
|
inherited |
References Spectrum::b_bounds, Origen::EMAX, and Origen::EMIN.
Referenced by EmissionSpectrum::energy_range().
|
inherited |
References Spectrum::total_energy_intensity(), and Spectrum::total_intensity().
|
inherited |
References Spectrum::newsp_energy_intensity().
Referenced by Spectrum::mean_energy(), and EmissionSpectrum::total_energy_intensity().
|
inherited |
References Spectrum::newptr_energy_intensity().
Referenced by Spectrum::total_energy_intensity().
|
inherited |
References Spectrum::b_bounds, Spectrum::b_intensity, and Spectrum::intensity_size().
Referenced by Spectrum::newsp_energy_intensity().
|
inherited |
return a shared (read-only) intensity pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
return a shared (read-only) bounds pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
Referenced by EmissionSpectrum::bin(), Spectrum::map_intensity(), and Spectrum::remap_intensity().
|
inherited |
References Spectrum::computeUnionWts_1(), and Spectrum::map_intensity().
|
staticinherited |
|
inherited |
|
inherited |
References Spectrum::b_intensity, Spectrum::b_new_bounds, Spectrum::b_union_grid, Spectrum::b_union_to_new, Spectrum::b_union_to_old, and Spectrum::b_union_wts.
Referenced by Spectrum::remap_intensity().
|
inherited |
|
inherited |
- Examples:
- tstDiscreteSpectrum.cpp, tstEmissionResource.cpp, tstEmissionSpectrum.cpp, tstGammaResource.cpp, and tstGammaResourceIO.cpp.
References Spectrum::b_bounds, and Spectrum::b_intensity.
Referenced by EmissionSpectrum::print_continuous(), TEST(), and TEST_P().
|
inherited |
get element of bounds vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
- Examples:
- tstGammaResourceIO.cpp.
Referenced by TEST().
|
inherited |
return view of bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by StateSetIO_s62b::write().
|
inherited |
return size of bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by Spectrum::canonicalize_intensity().
|
inherited |
return whether the class has a non-null bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
populate an existing Vec_Dbl with a copy of bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
set the bounds (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
retrieve pointer (read-only) to bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
get array of bounds vector (Fortran)
|
inherited |
set bounds vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
inherited |
get element of intensity vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
inherited |
return view of intensity vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by StateSetIO_s62b::write().
|
inherited |
return size of intensity vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by NeutronSpectra::is_consistent(), Spectrum::newptr_energy_intensity(), EmissionSpectrum::num_continuous(), and NeutronSpectra::resum().
|
inherited |
return whether the class has a non-null intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by Spectrum::total_intensity().
|
inherited |
populate an existing Vec_Dbl with a copy of intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
set the intensity (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
retrieve pointer (read-only) to intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
get array of intensity vector (Fortran)
|
inherited |
set intensity vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
staticinherited |
|
staticinherited |
Referenced by Spectrum::map_intensity().
|
protectedinherited |
|
protectedinherited |
References Spectrum::b_intensity, and Spectrum::bounds_size().
Member Data Documentation
|
protectedinherited |
|
protectedinherited |
The documentation for this type was generated from the following file:
- Core/dc/f/BetaSpectra_M.f90