#include <NeutronSpectra.h>
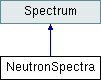
Container for gamma spectrum data. More...
Public Types | |
typedef std::shared_ptr< NeutronSpectra > | SP |
typedef std::shared_ptr< const NeutronSpectra > | SCP |
Typedefs | |
typedef std::function< SP_Vec_Dbl(const Vec_Dbl &, const Vec_Int &, const Vec_Dbl &)> | Integrator_t |
Basic Methods | |
const Vec_Dbl & | bounds () const |
return a const reference to bounds More... | |
void | set_bounds (const Vec_Dbl &) |
set the bounds (copy operation) More... | |
const Vec_Dbl & | intensity () const |
return a const reference to intensity More... | |
void | set_intensity (const Vec_Dbl &) |
set the intensity (copy operation) More... | |
Comparison | |
bool | match (const Spectrum &) const |
bool | operator== (const Spectrum &) const |
static bool | match (const Spectrum &, const Spectrum &) |
Convenience Methods | |
double | total_intensity () const |
EnergyRange_t | energy_range () const |
double | mean_energy () const |
double | total_energy_intensity () const |
SP_Vec_Dbl | newsp_energy_intensity () const |
Memory-usage Variants of Other Methods | |
Vec_Dbl * | newptr_energy_intensity () const |
SCP_Vec_Dbl | scp_intensity () const |
return a shared (read-only) intensity pointer More... | |
SCP_Vec_Dbl | scp_bounds () const |
return a shared (read-only) bounds pointer More... | |
Mapping Methods | |
bool | map_intensity (const Vec_Dbl &new_bounds, Integrator_t integrator, Vec_Dbl *intensity, double wt=1.0, double tol=ETOL) const |
bool | map_intensity (const Vec_Dbl &new_bounds, Vec_Dbl *intensity, double wt=1.0, double tol=ETOL) const |
void | remap_intensity (Vec_Dbl *intensity, double wt=1.0) const |
bool | has_mapping_variables () const |
void | clear_mapping_variables () const |
std::string | print_bins () const |
Additional Methods for Language Bindings (e.g. Fortran) | |
double | bounds_at (size_t) const |
get element of bounds vector by index More... | |
double const * | bounds_array () const |
return view of bounds vector More... | |
size_t | bounds_size () const |
return size of bounds vector More... | |
bool | has_bounds () const |
return whether the class has a non-null bounds More... | |
void | get_bounds (Vec_Dbl &) const |
populate an existing Vec_Dbl with a copy of bounds More... | |
void | manageptr_bounds (Vec_Dbl *&) |
set the bounds (ownership transfer) More... | |
void | getptr_bounds (Vec_Dbl const *&) const |
retrieve pointer (read-only) to bounds More... | |
void | get_bounds_array (size_t, double *) const |
get array of bounds vector (Fortran) More... | |
void | set_bounds_array (size_t, double *) |
set bounds vector from array (Fortran) More... | |
double | intensity_at (size_t) const |
get element of intensity vector by index More... | |
double const * | intensity_array () const |
return view of intensity vector More... | |
size_t | intensity_size () const |
return size of intensity vector More... | |
bool | has_intensity () const |
return whether the class has a non-null intensity More... | |
void | get_intensity (Vec_Dbl &) const |
populate an existing Vec_Dbl with a copy of intensity More... | |
void | manageptr_intensity (Vec_Dbl *&) |
set the intensity (ownership transfer) More... | |
void | getptr_intensity (Vec_Dbl const *&) const |
retrieve pointer (read-only) to intensity More... | |
void | get_intensity_array (size_t, double *) const |
get array of intensity vector (Fortran) More... | |
void | set_intensity_array (size_t, double *) |
set intensity vector from array (Fortran) More... | |
Static Helper Functions | |
returns true if the new and old bounds have the same min/max energy, i.e. the mapping will be conservative | |
static void | map_intensity (const Vec_Dbl &old_bounds, const Vec_Dbl &new_bounds, const Vec_Int &union_to_old, const Vec_Int &union_to_new, const Vec_Dbl &union_grid, const Vec_Dbl &old_intensity, const Vec_Dbl &union_wts, Vec_Dbl *new_intensity, double wt=1.0) |
static std::string | print_bins (const Vec_Dbl &bounds, const Vec_Dbl &intensity) |
static bool | map_bins (const Vec_Dbl &old_bounds, const Vec_Dbl &new_bounds, Vec_Int *union_to_old, Vec_Int *union_to_new, Vec_Dbl *union_grid, double tol=ETOL) |
static SP_Vec_Dbl | computeUnionWts_1 (const Vec_Dbl &union_grid, const Vec_Int &union_to_old, const Vec_Dbl &old_flux) |
Other | |
SP_Vec_Dbl | b_bounds |
SP_Vec_Dbl | b_intensity |
void | canonicalize_bounds () |
void | canonicalize_intensity () |
Public Member Functions | |
NeutronSpectra () | |
virtual | ~NeutronSpectra () |
bool | is_consistent () const |
void | resum () |
bool | match (const NeutronSpectra &) const |
bool | operator== (const NeutronSpectra &) const |
const Vec_Dbl & | intensity_sf () const |
return a const reference to intensity_sf More... | |
SCP_Vec_Dbl | scp_intensity_sf () const |
return a shared (read-only) intensity_sf pointer More... | |
double const * | intensity_sf_array () const |
return view of intensity_sf vector More... | |
bool | has_intensity_sf () const |
return whether the class has a non-null intensity_sf More... | |
void | set_intensity_sf (const Vec_Dbl &) |
set the intensity_sf (copy operation) More... | |
void | get_intensity_sf (Vec_Dbl &) const |
populate an existing Vec_Dbl with a copy of intensity_sf More... | |
void | manageptr_intensity_sf (Vec_Dbl *&) |
set the intensity_sf (ownership transfer) More... | |
void | getptr_intensity_sf (Vec_Dbl const *&) const |
retrieve pointer (read-only) to intensity_sf More... | |
void | get_intensity_sf_array (size_t, double *) const |
get array of intensity_sf vector (Fortran) More... | |
void | set_intensity_sf_array (size_t, double *) |
set intensity_sf vector from array (Fortran) More... | |
size_t | intensity_sf_size () const |
return size of intensity_sf vector More... | |
double | intensity_sf_at (size_t) const |
get element of intensity_sf vector by index More... | |
const Vec_Dbl & | intensity_alphan () const |
return a const reference to intensity_alphan More... | |
SCP_Vec_Dbl | scp_intensity_alphan () const |
return a shared (read-only) intensity_alphan pointer More... | |
double const * | intensity_alphan_array () const |
return view of intensity_alphan vector More... | |
bool | has_intensity_alphan () const |
return whether the class has a non-null intensity_alphan More... | |
void | set_intensity_alphan (const Vec_Dbl &) |
set the intensity_alphan (copy operation) More... | |
void | get_intensity_alphan (Vec_Dbl &) const |
populate an existing Vec_Dbl with a copy of intensity_alphan More... | |
void | manageptr_intensity_alphan (Vec_Dbl *&) |
set the intensity_alphan (ownership transfer) More... | |
void | getptr_intensity_alphan (Vec_Dbl const *&) const |
retrieve pointer (read-only) to intensity_alphan More... | |
void | get_intensity_alphan_array (size_t, double *) const |
get array of intensity_alphan vector (Fortran) More... | |
void | set_intensity_alphan_array (size_t, double *) |
set intensity_alphan vector from array (Fortran) More... | |
size_t | intensity_alphan_size () const |
return size of intensity_alphan vector More... | |
double | intensity_alphan_at (size_t) const |
get element of intensity_alphan vector by index More... | |
const Vec_Dbl & | intensity_delayn () const |
return a const reference to intensity_delayn More... | |
SCP_Vec_Dbl | scp_intensity_delayn () const |
return a shared (read-only) intensity_delayn pointer More... | |
double const * | intensity_delayn_array () const |
return view of intensity_delayn vector More... | |
bool | has_intensity_delayn () const |
return whether the class has a non-null intensity_delayn More... | |
void | set_intensity_delayn (const Vec_Dbl &) |
set the intensity_delayn (copy operation) More... | |
void | get_intensity_delayn (Vec_Dbl &) const |
populate an existing Vec_Dbl with a copy of intensity_delayn More... | |
void | manageptr_intensity_delayn (Vec_Dbl *&) |
set the intensity_delayn (ownership transfer) More... | |
void | getptr_intensity_delayn (Vec_Dbl const *&) const |
retrieve pointer (read-only) to intensity_delayn More... | |
void | get_intensity_delayn_array (size_t, double *) const |
get array of intensity_delayn vector (Fortran) More... | |
void | set_intensity_delayn_array (size_t, double *) |
set intensity_delayn vector from array (Fortran) More... | |
size_t | intensity_delayn_size () const |
return size of intensity_delayn vector More... | |
double | intensity_delayn_at (size_t) const |
get element of intensity_delayn vector by index More... | |
Static Public Member Functions | |
static bool | match (const NeutronSpectra &, const NeutronSpectra &) |
Protected Member Functions | |
void | canonicalize_intensity_sf () |
void | canonicalize_intensity_alphan () |
void | canonicalize_intensity_delayn () |
Protected Attributes | |
SP_Vec_Dbl | d_intensity_sf |
SP_Vec_Dbl | d_intensity_alphan |
SP_Vec_Dbl | d_intensity_delayn |
Detailed Description
Container for gamma spectrum data.
The NeutronSpectra data container manages the gamma intensity and the group structure.
- Examples:
- tstNeutronSpectra.cpp.
Member Typedef Documentation
typedef std::shared_ptr<NeutronSpectra> SP |
typedef std::shared_ptr<const NeutronSpectra> SCP |
|
inherited |
Constructor & Destructor Documentation
NeutronSpectra | ( | ) |
|
inlinevirtual |
Member Function Documentation
bool is_consistent | ( | ) | const |
References Spectrum::b_intensity, NeutronSpectra::d_intensity_alphan, NeutronSpectra::d_intensity_delayn, NeutronSpectra::d_intensity_sf, NeutronSpectra::has_intensity_alphan(), NeutronSpectra::has_intensity_delayn(), NeutronSpectra::has_intensity_sf(), NeutronSpectra::intensity_alphan_size(), NeutronSpectra::intensity_delayn_size(), NeutronSpectra::intensity_sf_size(), and Spectrum::intensity_size().
void resum | ( | ) |
|
static |
References Spectrum::match().
Referenced by NeutronSpectra::match().
bool match | ( | const NeutronSpectra & | b | ) | const |
References NeutronSpectra::match().
bool operator== | ( | const NeutronSpectra & | b | ) | const |
const Vec_Dbl & intensity_sf | ( | ) | const |
return a const reference to intensity_sf
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of NeutronSpectra goes out of scope.
Referenced by StateSetIO_bof::write().
SCP_Vec_Dbl scp_intensity_sf | ( | ) | const |
return a shared (read-only) intensity_sf pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Vec_Dbl::value_type const * intensity_sf_array | ( | ) | const |
return view of intensity_sf vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by StateSetIO_s62b::write().
bool has_intensity_sf | ( | ) | const |
return whether the class has a non-null intensity_sf
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by NeutronSpectra::is_consistent(), NeutronSpectra::operator==(), NeutronSpectra::resum(), and StateSetIO_bof::write().
void set_intensity_sf | ( | const Vec_Dbl & | intensity_sf | ) |
set the intensity_sf (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by FakeFactory::newspStateSet_10nuclide(), StateSetIO_s62b::read(), StateSetIO_s61::read(), StateSetIO_bof::read(), and FakeFactory::StateSet_random1().
void get_intensity_sf | ( | Vec_Dbl & | intensity_sf | ) | const |
populate an existing Vec_Dbl with a copy of intensity_sf
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void manageptr_intensity_sf | ( | Vec_Dbl *& | intensity_sf | ) |
set the intensity_sf (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void getptr_intensity_sf | ( | Vec_Dbl const *& | intensity_sf | ) | const |
retrieve pointer (read-only) to intensity_sf
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_intensity_sf_array | ( | size_t | , |
double * | |||
) | const |
get array of intensity_sf vector (Fortran)
void set_intensity_sf_array | ( | size_t | , |
double * | |||
) |
set intensity_sf vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
size_t intensity_sf_size | ( | ) | const |
return size of intensity_sf vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by NeutronSpectra::is_consistent().
Vec_Dbl::value_type intensity_sf_at | ( | size_t | i | ) | const |
get element of intensity_sf vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
const Vec_Dbl & intensity_alphan | ( | ) | const |
return a const reference to intensity_alphan
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of NeutronSpectra goes out of scope.
Referenced by StateSetIO_bof::write().
SCP_Vec_Dbl scp_intensity_alphan | ( | ) | const |
return a shared (read-only) intensity_alphan pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Vec_Dbl::value_type const * intensity_alphan_array | ( | ) | const |
return view of intensity_alphan vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by StateSetIO_s62b::write().
bool has_intensity_alphan | ( | ) | const |
return whether the class has a non-null intensity_alphan
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by NeutronSpectra::is_consistent(), NeutronSpectra::operator==(), NeutronSpectra::resum(), and StateSetIO_bof::write().
void set_intensity_alphan | ( | const Vec_Dbl & | intensity_alphan | ) |
set the intensity_alphan (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by FakeFactory::newspStateSet_10nuclide(), StateSetIO_s62b::read(), StateSetIO_s61::read(), StateSetIO_bof::read(), and FakeFactory::StateSet_random1().
void get_intensity_alphan | ( | Vec_Dbl & | intensity_alphan | ) | const |
populate an existing Vec_Dbl with a copy of intensity_alphan
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void manageptr_intensity_alphan | ( | Vec_Dbl *& | intensity_alphan | ) |
set the intensity_alphan (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void getptr_intensity_alphan | ( | Vec_Dbl const *& | intensity_alphan | ) | const |
retrieve pointer (read-only) to intensity_alphan
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_intensity_alphan_array | ( | size_t | , |
double * | |||
) | const |
get array of intensity_alphan vector (Fortran)
void set_intensity_alphan_array | ( | size_t | , |
double * | |||
) |
set intensity_alphan vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
size_t intensity_alphan_size | ( | ) | const |
return size of intensity_alphan vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by NeutronSpectra::is_consistent().
Vec_Dbl::value_type intensity_alphan_at | ( | size_t | i | ) | const |
get element of intensity_alphan vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
const Vec_Dbl & intensity_delayn | ( | ) | const |
return a const reference to intensity_delayn
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of NeutronSpectra goes out of scope.
Referenced by StateSetIO_bof::write().
SCP_Vec_Dbl scp_intensity_delayn | ( | ) | const |
return a shared (read-only) intensity_delayn pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Vec_Dbl::value_type const * intensity_delayn_array | ( | ) | const |
return view of intensity_delayn vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by StateSetIO_s62b::write().
bool has_intensity_delayn | ( | ) | const |
return whether the class has a non-null intensity_delayn
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by NeutronSpectra::is_consistent(), NeutronSpectra::operator==(), NeutronSpectra::resum(), and StateSetIO_bof::write().
void set_intensity_delayn | ( | const Vec_Dbl & | intensity_delayn | ) |
set the intensity_delayn (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by FakeFactory::newspStateSet_10nuclide(), StateSetIO_s62b::read(), StateSetIO_bof::read(), and FakeFactory::StateSet_random1().
void get_intensity_delayn | ( | Vec_Dbl & | intensity_delayn | ) | const |
populate an existing Vec_Dbl with a copy of intensity_delayn
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void manageptr_intensity_delayn | ( | Vec_Dbl *& | intensity_delayn | ) |
set the intensity_delayn (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void getptr_intensity_delayn | ( | Vec_Dbl const *& | intensity_delayn | ) | const |
retrieve pointer (read-only) to intensity_delayn
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_intensity_delayn_array | ( | size_t | , |
double * | |||
) | const |
get array of intensity_delayn vector (Fortran)
void set_intensity_delayn_array | ( | size_t | , |
double * | |||
) |
set intensity_delayn vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
size_t intensity_delayn_size | ( | ) | const |
return size of intensity_delayn vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by NeutronSpectra::is_consistent().
Vec_Dbl::value_type intensity_delayn_at | ( | size_t | i | ) | const |
get element of intensity_delayn vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
protected |
|
protected |
|
protected |
|
inherited |
return a const reference to bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of Spectrum goes out of scope.
Referenced by Spectrum::operator==(), and StateSetIO_bof::write().
|
inherited |
set the bounds (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
- Examples:
- tstEmissionResource.cpp, tstEmissionSpectrum.cpp, and tstGammaResource.cpp.
Referenced by FakeFactory::newspStateSet_10nuclide(), StateSetIO_s62b::read(), GammaResourceIO_cimg::read(), StateSetIO_bof::read(), StateSetIO_s61::read(), FakeFactory::StateSet_random1(), and TEST().
|
inherited |
return a const reference to intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of Spectrum goes out of scope.
Referenced by Spectrum::operator==(), and StateSetIO_bof::write().
|
inherited |
set the intensity (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by FakeFactory::newspStateSet_10nuclide(), StateSetIO_s62b::read(), GammaResourceIO_cimg::read(), StateSetIO_bof::read(), StateSetIO_s61::read(), and FakeFactory::StateSet_random1().
|
inherited |
Referenced by NeutronSpectra::match(), AlphaSpectra::match(), GammaSpectra::match(), and BetaSpectra::match().
References Spectrum::b_bounds.
|
inherited |
References Spectrum::b_bounds, Spectrum::b_intensity, Spectrum::bounds(), and Spectrum::intensity().
Referenced by AlphaSpectra::operator==(), BetaSpectra::operator==(), and GammaSpectra::operator==().
|
inherited |
References Spectrum::b_intensity, and Spectrum::has_intensity().
Referenced by Spectrum::mean_energy(), and EmissionSpectrum::total_intensity().
|
inherited |
References Spectrum::b_bounds, Origen::EMAX, and Origen::EMIN.
Referenced by EmissionSpectrum::energy_range().
|
inherited |
References Spectrum::total_energy_intensity(), and Spectrum::total_intensity().
|
inherited |
References Spectrum::newsp_energy_intensity().
Referenced by Spectrum::mean_energy(), and EmissionSpectrum::total_energy_intensity().
|
inherited |
References Spectrum::newptr_energy_intensity().
Referenced by Spectrum::total_energy_intensity().
|
inherited |
References Spectrum::b_bounds, Spectrum::b_intensity, and Spectrum::intensity_size().
Referenced by Spectrum::newsp_energy_intensity().
|
inherited |
return a shared (read-only) intensity pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
return a shared (read-only) bounds pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
Referenced by EmissionSpectrum::bin(), Spectrum::map_intensity(), and Spectrum::remap_intensity().
|
inherited |
References Spectrum::computeUnionWts_1(), and Spectrum::map_intensity().
|
staticinherited |
|
inherited |
|
inherited |
References Spectrum::b_intensity, Spectrum::b_new_bounds, Spectrum::b_union_grid, Spectrum::b_union_to_new, Spectrum::b_union_to_old, and Spectrum::b_union_wts.
Referenced by Spectrum::remap_intensity().
|
inherited |
|
inherited |
- Examples:
- tstDiscreteSpectrum.cpp, tstEmissionResource.cpp, tstEmissionSpectrum.cpp, tstGammaResource.cpp, and tstGammaResourceIO.cpp.
References Spectrum::b_bounds, and Spectrum::b_intensity.
Referenced by EmissionSpectrum::print_continuous(), TEST(), and TEST_P().
|
inherited |
get element of bounds vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
- Examples:
- tstGammaResourceIO.cpp.
Referenced by TEST().
|
inherited |
return view of bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by StateSetIO_s62b::write().
|
inherited |
return size of bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by Spectrum::canonicalize_intensity().
|
inherited |
return whether the class has a non-null bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
populate an existing Vec_Dbl with a copy of bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
set the bounds (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
retrieve pointer (read-only) to bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
get array of bounds vector (Fortran)
|
inherited |
set bounds vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
inherited |
get element of intensity vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
inherited |
return view of intensity vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by StateSetIO_s62b::write().
|
inherited |
return size of intensity vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by NeutronSpectra::is_consistent(), Spectrum::newptr_energy_intensity(), EmissionSpectrum::num_continuous(), and NeutronSpectra::resum().
|
inherited |
return whether the class has a non-null intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by Spectrum::total_intensity().
|
inherited |
populate an existing Vec_Dbl with a copy of intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
set the intensity (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
retrieve pointer (read-only) to intensity
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
inherited |
get array of intensity vector (Fortran)
|
inherited |
set intensity vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
staticinherited |
|
staticinherited |
Referenced by Spectrum::map_intensity().
|
protectedinherited |
|
protectedinherited |
References Spectrum::b_intensity, and Spectrum::bounds_size().
Member Data Documentation
|
protected |
Vec_Dbl - spontaneous fission spectrum (num_neutron_groups)
Referenced by NeutronSpectra::is_consistent(), NeutronSpectra::operator==(), and NeutronSpectra::resum().
|
protected |
Vec_Dbl - (alpha,n) spectrum (num_neutron_groups)
Referenced by NeutronSpectra::is_consistent(), NeutronSpectra::operator==(), and NeutronSpectra::resum().
|
protected |
Vec_Dbl - delayed neutron spectrum (num_neutron_groups)
Referenced by NeutronSpectra::is_consistent(), NeutronSpectra::operator==(), and NeutronSpectra::resum().
|
protectedinherited |
|
protectedinherited |
The documentation for this class was generated from the following files:
- Core/dc/NeutronSpectra.h
- Core/dc/NeutronSpectra.cpp