#include <Nuclide_Gen.h>
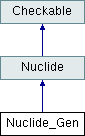
General-purpose implementation of the Nuclide interface. More...
Public Types | |
typedef SP_Nuclide | SP |
strong pointer More... | |
typedef WP_Nuclide | WP |
weak pointer More... | |
typedef CWP_Nuclide | CWP |
const weak pointer More... | |
INTERFACE<<Origen::Checkable>> | |
See Origen::Checkable for details. | |
std::string | name () const |
void | get_name (std::string &name) const |
void | set_name (std::string name) |
int | id () const |
void | get_id (int &id) const |
void | set_id (int id) |
bool | has_initialized_id () const |
std::string | to_string () const |
bool | good () const |
bool | good (Vec_Str &errors) const |
bool | is_initialized () const |
Scale::Json::Value | to_json () const |
INTERFACE<<Origen::Nuclide>> | |
See Origen::Nuclide for details. | |
std::vector< WP_Transition > | gains () const |
Return a vector of gains producing the nuclide. More... | |
void | get_gains (std::vector< WP_Transition > &gains) const |
Get a vector of gains producing the nuclide. More... | |
void | set_gains (std::vector< WP_Transition > gains) |
Set the gains producing the nuclide. More... | |
void | append_gain (WP_Transition gain) |
Append gain to list of gains. More... | |
void | clear_gains () |
WP_Transition | gain (int channel_id, int parent_id) const |
retrieve a gain More... | |
int | num_gains () const |
Get the number of gains producing the nuclide. More... | |
std::vector< SP_Channel > | losses () const |
Return a vector of losses destroying the nuclide. More... | |
void | get_losses (std::vector< SP_Channel > &losses) const |
Get a vector of losses destroying the nuclide. More... | |
void | set_losses (std::vector< SP_Channel > losses) |
Set the losses destroying the nuclide. More... | |
SP_Channel | loss (int channel_id) const |
return loss channel More... | |
void | get_loss (int channel_id, SP_Channel &loss) const |
retrieve loss channel More... | |
void | set_loss (SP_Channel loss) |
SP_Channel | add_loss (SP_ChannelType channel_type) |
add a new loss channel to the nuclide by specifying ChannelType More... | |
SP_Channel | add_loss (int field_id, int reaction_mt, int decay_mode=0) |
add a new loss channel by specifying raw ids More... | |
SP_Channel | add_loss (SP_Field field, int reaction_mt, int decay_mode=0) |
add a new loss channel with Field and raw ids More... | |
SP_Channel | add_loss (int decay_mode) |
add a decay loss More... | |
int | num_losses () const |
Get the number of losses destroying the nuclide. More... | |
CWP_TransitionSystem | system () const |
void | get_system (WP_TransitionSystem &system) const |
void | set_system (WP_TransitionSystem system) |
bool | has_initialized_system () const |
int | isomeric_state () const |
void | get_isomeric_state (int &isomeric_state) const |
void | set_isomeric_state (int isomeric_state) |
SP_Species | species () const |
void | get_species (SP_Species &species) const |
void | set_species (SP_Species species) |
bool | has_initialized_species () const |
double | num () const |
void | get_num (double &num) const |
void | set_num (double num) |
double | decay_constant () const |
Return the decay constant. More... | |
double | loss_xs () const |
Public Member Functions | |
Nuclide_Gen () | |
Nuclide_Gen (int isomeric_state, Species::SP species) | |
Nuclide_Gen (int isomeric_state, Species::SP species, double num) | |
virtual | ~Nuclide_Gen () |
Private Types | |
typedef std::map< int, Channel::SP > | ChannelRegistry |
Private Member Functions | |
virtual std::vector< WP_Transition > | gains_impl () const |
virtual void | get_gains_impl (std::vector< WP_Transition > &gains) const |
virtual void | set_gains_impl (std::vector< WP_Transition > gains) |
virtual int | num_gains_impl () const |
virtual void | append_gain_impl (WP_Transition gain) |
virtual WP_Transition | gain_impl (int channel_id, int parent_id) const |
virtual void | clear_gains_impl () |
virtual std::vector< Channel::SP > | losses_impl () const |
virtual void | get_losses_impl (std::vector< Channel::SP > &losses) const |
virtual void | set_losses_impl (std::vector< Channel::SP > losses) |
virtual Channel::SP | loss_impl (int channel_id) const |
virtual void | get_loss_impl (int channel_id, Channel::SP &loss) const |
virtual void | set_loss_impl (Channel::SP loss) |
virtual SP_Channel | add_loss_impl (SP_ChannelType channel_type) |
virtual int | num_losses_impl () const |
virtual double | decay_constant_impl () const |
virtual double | num_impl () const |
virtual void | get_num_impl (double &num) const |
virtual void | set_num_impl (double num) |
virtual int | isomeric_state_impl () const |
virtual void | get_isomeric_state_impl (int &isomeric_state) const |
virtual void | set_isomeric_state_impl (int isomeric_state) |
virtual double | loss_xs_impl () const |
virtual Species::SP | species_impl () const |
virtual void | get_species_impl (Species::SP &species) const |
virtual void | set_species_impl (Species::SP species) |
virtual bool | has_initialized_species_impl () const |
virtual CWP_TransitionSystem | system_impl () const |
virtual void | get_system_impl (WP_TransitionSystem &system) const |
virtual void | set_system_impl (WP_TransitionSystem system) |
virtual bool | has_initialized_system_impl () const |
Private Attributes | |
TransitionSystem::WP | b_system |
int | b_isomeric_state |
Species::SP | b_species |
double | b_num |
std::vector< WP_Transition > | b_gains |
ChannelRegistry | b_loss_map |
Detailed Description
General-purpose implementation of the Nuclide interface.
The general-purpose implementation of INTERFACE<<Origen::Nuclide>> that will be good when the use case is:
- an initial intense setup operation adding loss channels followed by
- repeated access losses without changing structure and
- extraction of all gains in a group.
The gains are stored in a vector so the gain lookup is linear, whereas the losses are stored in a map.
Sometimes the best approach is to store the smart pointers to what you need in a structure that you can easily/quickly traverse.
- Examples:
- tstTransitionSystem_Gen.cpp.
Member Typedef Documentation
|
private |
|
inherited |
strong pointer
|
inherited |
weak pointer
|
inherited |
const weak pointer
Constructor & Destructor Documentation
Nuclide_Gen | ( | ) |
Nuclide_Gen | ( | int | isomeric_state, |
Species::SP | species | ||
) |
References Nuclide::set_isomeric_state(), and Nuclide::set_species().
Nuclide_Gen | ( | int | isomeric_state, |
Species::SP | species, | ||
double | num | ||
) |
References Nuclide::set_isomeric_state(), Nuclide::set_num(), and Nuclide::set_species().
|
virtual |
Member Function Documentation
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_gains.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_gains.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_gains, and Nuclide::gains().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_gains.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_gains, Transition::channel(), and Channel::parent().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_gains, Checkable::id(), and Channel::parent().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_gains, and Nuclide::num_gains().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map, and Nuclide::losses().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map, Checkable::has_initialized_id(), and Checkable::id().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map.
|
privatevirtual |
Implements Nuclide.
References Nuclide::loss().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map, and Nuclide::loss().
|
privatevirtual |
Implements Nuclide.
References Nuclide::loss(), and Nuclide::set_loss().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_num.
|
privatevirtual |
Implements Nuclide.
References Nuclide::num().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_num, and Nuclide::num().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_isomeric_state.
|
privatevirtual |
Implements Nuclide.
References Nuclide::isomeric_state().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_isomeric_state, and Nuclide::isomeric_state().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_loss_map.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_species.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_species.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_species, and Nuclide::species().
|
privatevirtual |
Implements Nuclide.
References Nuclide::species().
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_system.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_system.
|
privatevirtual |
Implements Nuclide.
References Nuclide_Gen::b_system, and Nuclide::system().
|
privatevirtual |
Implements Nuclide.
References Nuclide::system().
|
inherited |
Return a vector of gains producing the nuclide.
- Returns
- std::vector<WP_Transition> vector of gains.
Referenced by Nuclide_Gen::set_gains_impl().
|
inherited |
Get a vector of gains producing the nuclide.
The user vector is overwritten with the contents.
- Returns
- std::vector<WP_Transition> vector of gains.
|
inherited |
Set the gains producing the nuclide.
The internal gains are overwritten with the contents.
- Parameters
-
std::vector<WP_Transition> vector of gains.
|
inherited |
Append gain to list of gains.
- Parameters
-
gain to append.
|
inherited |
|
inherited |
retrieve a gain
|
inherited |
Get the number of gains producing the nuclide.
- Returns
- int number of gains.
Referenced by Nuclide_Gen::clear_gains_impl().
|
inherited |
Return a vector of losses destroying the nuclide.
- Returns
- std::vector<Channel::SP> vector of losses.
Referenced by Nuclide_Gen::losses_impl().
|
inherited |
Get a vector of losses destroying the nuclide.
The user vector is overwritten with the contents.
- Returns
- std::vector<Channel::SP> vector of losses.
|
inherited |
Set the losses destroying the nuclide.
The internal losses are overwritten with the contents.
- Returns
- std::vector<Channel::SP> vector of losses.
|
inherited |
return loss channel
Referenced by Nuclide_Gen::add_loss_impl(), Nuclide_Gen::get_loss_impl(), and Nuclide_Gen::set_loss_impl().
|
inherited |
retrieve loss channel
|
inherited |
Referenced by Nuclide_Gen::add_loss_impl().
|
inherited |
add a new loss channel to the nuclide by specifying ChannelType
|
inherited |
add a new loss channel by specifying raw ids
|
inherited |
add a new loss channel with Field and raw ids
|
inherited |
add a decay loss
|
inherited |
Get the number of losses destroying the nuclide.
- Returns
- int number of losses.
|
inherited |
Referenced by Nuclide_Gen::has_initialized_system_impl(), and Nuclide_Gen::set_system_impl().
|
inherited |
Referenced by Channel_Gen::add_transition_impl(), and Channel_Gen::update_byproduct_transitions_impl().
|
inherited |
|
inherited |
Referenced by Channel_Gen::add_transition_impl().
|
inherited |
Referenced by Nuclide_Gen::get_isomeric_state_impl(), and Nuclide_Gen::set_isomeric_state_impl().
|
inherited |
|
inherited |
Referenced by Nuclide_Gen::Nuclide_Gen().
|
inherited |
Referenced by Nuclide_Gen::has_initialized_species_impl(), and Nuclide_Gen::set_species_impl().
|
inherited |
|
inherited |
Referenced by Nuclide_Gen::Nuclide_Gen().
|
inherited |
|
inherited |
Referenced by Nuclide_Gen::get_num_impl(), Channel_Gen::rate_impl(), and Nuclide_Gen::set_num_impl().
|
inherited |
|
inherited |
Referenced by Nuclide_Gen::Nuclide_Gen().
|
inherited |
Return the decay constant.
This total decay constant is a sum of all channel decay constants.
- Returns
- double decay constant (1/s)
|
inherited |
|
inherited |
|
inherited |
- Examples:
- tstTransitionSystem_Gen.cpp.
Referenced by TEST().
|
inherited |
- Examples:
- tstTransitionSystem_Gen.cpp.
Referenced by TEST().
|
inherited |
- Examples:
- tstTransitionSystem_Gen.cpp.
Referenced by TransitionSystem_Gen::add_nuclide_impl(), TransitionSystem_Gen::add_species_impl(), Channel_Gen::add_transition_impl(), LibraryBuilder::create_decay_transitions(), LibraryBuilder::create_reaction_transitions(), Nuclide_Gen::gain_impl(), LibraryBuilder::search_reaction_transitions(), Nuclide_Gen::set_losses_impl(), Channel_Gen::set_transition_impl(), TEST(), and TEST_F().
|
inherited |
- Examples:
- tstTransitionSystem_Gen.cpp.
Referenced by TEST().
|
inherited |
- Examples:
- tstTransitionSystem_Gen.cpp.
Referenced by TEST().
|
inherited |
|
inherited |
- Examples:
- tstTransitionSystemAdapter_AmpxN.cpp.
Referenced by TEST_F().
|
inherited |
|
inherited |
|
inherited |
Referenced by ChannelType_Decay::good_impl(), and ChannelType_Decay::update_byproducts().
|
inherited |
Member Data Documentation
|
private |
Referenced by Nuclide_Gen::get_system_impl(), Nuclide_Gen::set_system_impl(), and Nuclide_Gen::system_impl().
|
private |
Referenced by Nuclide_Gen::isomeric_state_impl(), and Nuclide_Gen::set_isomeric_state_impl().
|
private |
Referenced by Nuclide_Gen::get_species_impl(), Nuclide_Gen::set_species_impl(), and Nuclide_Gen::species_impl().
|
private |
Referenced by Nuclide_Gen::num_impl(), and Nuclide_Gen::set_num_impl().
|
private |
|
private |
The documentation for this class was generated from the following files:
- Core/ts/Nuclide_Gen.h
- Core/ts/Nuclide_Gen.cpp