#include <ReactionResource.h>
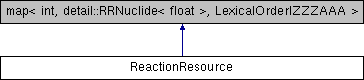
Multi-group cross section/yields resource. More...
Public Types | |
typedef detail::RRNuclide< float > | Nuclide |
typedef std::map< int, bool > | YieldNuclides_t |
typedef std::map< int, bool > | AllowedMts_t |
typedef std::shared_ptr< ReactionResource > | SP |
typedef std::shared_ptr< const ReactionResource > | SCP |
typedef std::map< int, std::pair< int, int > > | DirectYields_t |
Public Member Functions | |
ReactionResource () | |
ReactionResource (const Vec_Flt &storage_bounds) | |
virtual | ~ReactionResource () |
virtual FieldId | field_id () const |
field id for this data set, e.g. NEUTRON_FIELD More... | |
virtual void | print (std::ostream &os=std::cout) const |
print contents More... | |
virtual void | set_max_isomeric_state (int i) |
set max isomeric state More... | |
virtual int | max_isomeric_state () const |
get max isomeric state More... | |
virtual void | set_allowed_mts (const Vec_Int &allowed_mts, bool union_with=false) |
set allowed mts More... | |
virtual void | set_disallowed_mts (const Vec_Int &disallowed_mts, bool union_with=true) |
set disallowed mts More... | |
virtual void | get_allowed_mts (Vec_Int *allowed_mts) const |
get allowed mts More... | |
virtual void | get_disallowed_mts (Vec_Int *disallowed_mts) const |
get disallowed mts More... | |
virtual const AllowedMts_t & | allowed_mts () const |
direct return of allowed mts More... | |
virtual void | set_yield_nuclides (const Vec_Int &nuclides, bool union_with=false) |
set nuclides with allowed fission yields (used only in import) More... | |
virtual void | get_yield_nuclides (Vec_Int *nuclides) const |
get nuclides with allowed fission yields (used only in import) More... | |
virtual const YieldNuclides_t & | yield_nuclides () const |
direct return of allowed fission yields (used only in import) More... | |
virtual const DirectYields_t & | direct_yields () const |
direct yields More... | |
virtual void | set_direct_yields (int mt, int target_mt, int izzzaaa) |
set new direct yields More... | |
virtual void | delete_direct_yields (int mt) |
remove direct yields More... | |
void | import_fission_yields (const YieldResource &yr) |
const Vec_Flt & | storage_bounds () const |
return a const reference to storage_bounds More... | |
float | storage_bounds_at (size_t) const |
get element of storage_bounds vector by index More... | |
SCP_Vec_Flt | scp_storage_bounds () const |
return a shared (read-only) storage_bounds pointer More... | |
float const * | storage_bounds_array () const |
return view of storage_bounds vector More... | |
size_t | storage_bounds_size () const |
return size of storage_bounds vector More... | |
bool | has_storage_bounds () const |
return whether the class has a non-null storage_bounds More... | |
void | set_storage_bounds (const Vec_Flt &) |
set the storage_bounds (copy operation) More... | |
void | get_storage_bounds (Vec_Flt &) const |
populate an existing Vec_Flt with a copy of storage_bounds More... | |
void | manageptr_storage_bounds (Vec_Flt *&) |
set the storage_bounds (ownership transfer) More... | |
void | getptr_storage_bounds (Vec_Flt const *&) const |
retrieve pointer (read-only) to storage_bounds More... | |
void | get_storage_bounds_array (size_t, float *) const |
get array of storage_bounds vector (Fortran) More... | |
void | set_storage_bounds_array (size_t, float *) |
set storage_bounds vector from array (Fortran) More... | |
const Vec_Flt & | storage_flux () const |
return a const reference to storage_flux More... | |
float | storage_flux_at (size_t) const |
get element of storage_flux vector by index More... | |
SCP_Vec_Flt | scp_storage_flux () const |
return a shared (read-only) storage_flux pointer More... | |
float const * | storage_flux_array () const |
return view of storage_flux vector More... | |
size_t | storage_flux_size () const |
return size of storage_flux vector More... | |
bool | has_storage_flux () const |
return whether the class has a non-null storage_flux More... | |
void | get_storage_flux (Vec_Flt &) const |
populate an existing Vec_Flt with a copy of storage_flux More... | |
void | getptr_storage_flux (Vec_Flt const *&) const |
retrieve pointer (read-only) to storage_flux More... | |
void | get_storage_flux_array (size_t, float *) const |
get array of storage_flux vector (Fortran) More... | |
const Vec_Flt & | library_bounds () const |
return a const reference to library_bounds More... | |
float | library_bounds_at (size_t) const |
get element of library_bounds vector by index More... | |
SCP_Vec_Flt | scp_library_bounds () const |
return a shared (read-only) library_bounds pointer More... | |
float const * | library_bounds_array () const |
return view of library_bounds vector More... | |
size_t | library_bounds_size () const |
return size of library_bounds vector More... | |
bool | has_library_bounds () const |
return whether the class has a non-null library_bounds More... | |
void | set_library_bounds (const Vec_Flt &) |
set the library_bounds (copy operation) More... | |
void | get_library_bounds (Vec_Flt &) const |
populate an existing Vec_Flt with a copy of library_bounds More... | |
void | manageptr_library_bounds (Vec_Flt *&) |
set the library_bounds (ownership transfer) More... | |
void | getptr_library_bounds (Vec_Flt const *&) const |
retrieve pointer (read-only) to library_bounds More... | |
void | get_library_bounds_array (size_t, float *) const |
get array of library_bounds vector (Fortran) More... | |
void | set_library_bounds_array (size_t, float *) |
set library_bounds vector from array (Fortran) More... | |
const Vec_Flt & | library_flux () const |
return a const reference to library_flux More... | |
float | library_flux_at (size_t) const |
get element of library_flux vector by index More... | |
SCP_Vec_Flt | scp_library_flux () const |
return a shared (read-only) library_flux pointer More... | |
float const * | library_flux_array () const |
return view of library_flux vector More... | |
size_t | library_flux_size () const |
return size of library_flux vector More... | |
bool | has_library_flux () const |
return whether the class has a non-null library_flux More... | |
void | set_library_flux (const Vec_Flt &) |
set the library_flux (copy operation) More... | |
void | get_library_flux (Vec_Flt &) const |
populate an existing Vec_Flt with a copy of library_flux More... | |
void | manageptr_library_flux (Vec_Flt *&) |
set the library_flux (ownership transfer) More... | |
void | getptr_library_flux (Vec_Flt const *&) const |
retrieve pointer (read-only) to library_flux More... | |
void | get_library_flux_array (size_t, float *) const |
get array of library_flux vector (Fortran) More... | |
void | set_library_flux_array (size_t, float *) |
set library_flux vector from array (Fortran) More... | |
void | map_xs (Vec_Flt *xs) const |
void | map_flux (Vec_Flt *flux) const |
bool | using_data_mapper () const |
Public Attributes | |
K | keys |
STL member. More... | |
T | elements |
STL member. More... | |
Protected Member Functions | |
void | set_storage_flux (const Vec_Flt &) |
set the storage_flux (copy operation) More... | |
void | manageptr_storage_flux (Vec_Flt *&) |
set the storage_flux (ownership transfer) More... | |
void | set_storage_flux_array (size_t, float *) |
set storage_flux vector from array (Fortran) More... | |
void | canonicalize_storage_bounds () |
void | canonicalize_storage_flux () |
void | canonicalize_library_bounds () |
void | canonicalize_library_flux () |
Static Protected Member Functions | |
static void | default_allowed_mts (AllowedMts_t &allowed_mts) |
static void | default_isomeric_states (int max_isomeric_state, AllowedMts_t &allowed_mts) |
static void | default_direct_yields (DirectYields_t &direct_yields) |
Protected Attributes | |
SP_Vec_Flt | d_storage_bounds |
int | d_max_isomeric_state |
bool | d_using_data_mapper |
SP_Vec_Flt | d_storage_flux |
SP_Vec_Flt | d_library_flux |
SP_Vec_Flt | d_library_bounds |
ScaleData::MGXS1DMapper< float > | d_data_mapper |
YieldNuclides_t | d_yield_nuclides |
AllowedMts_t | d_allowed_mts |
DirectYields_t | d_direct_yields |
Detailed Description
Multi-group cross section/yields resource.
ReactionResource uses is a simple map of nuclide ids to ReactionResource::Nuclide, a sub-class used to manage ReactionResource::Nuclide::Xs, multi-group cross section and yields data.
Member Typedef Documentation
typedef detail::RRNuclide<float> Nuclide |
typedef std::map<int, bool> YieldNuclides_t |
typedef std::map<int, bool> AllowedMts_t |
typedef std::shared_ptr<ReactionResource> SP |
typedef std::shared_ptr<const ReactionResource> SCP |
typedef std::map<int, std::pair<int, int> > DirectYields_t |
Constructor & Destructor Documentation
ReactionResource | ( | ) |
ReactionResource | ( | const Vec_Flt & | storage_bounds | ) |
|
inlinevirtual |
Member Function Documentation
|
virtual |
field id for this data set, e.g. NEUTRON_FIELD
References Origen::NEUTRON_FIELD.
|
virtual |
print contents
- Examples:
- tstTransitionMatrixUpdater.cpp.
References RRXs< T >::dyield, RRXs< T >::iyield, RRXs< T >::pyield, RRXs< T >::totxs, RRXs< T >::yield_type, and Origen::yield_type_name().
Referenced by Origen::test::TEST().
|
virtual |
set max isomeric state
References ReactionResource::d_max_isomeric_state.
|
virtual |
get max isomeric state
References ReactionResource::d_max_isomeric_state.
Referenced by ReactionResource::default_isomeric_states(), and Origen::populateReactionResource_AmpxLibrary().
|
virtual |
set allowed mts
References ReactionResource::d_allowed_mts.
|
virtual |
set disallowed mts
References ReactionResource::d_allowed_mts.
Referenced by Origen::populateReactionResource_AmpxLibrary().
|
virtual |
get allowed mts
References ReactionResource::d_allowed_mts.
|
virtual |
get disallowed mts
References ReactionResource::d_allowed_mts.
|
virtual |
direct return of allowed mts
References ReactionResource::d_allowed_mts.
Referenced by Origen::populateReactionResource_AmpxLibrary().
|
virtual |
set nuclides with allowed fission yields (used only in import)
References ReactionResource::d_yield_nuclides.
|
virtual |
get nuclides with allowed fission yields (used only in import)
References ReactionResource::d_yield_nuclides.
|
virtual |
direct return of allowed fission yields (used only in import)
References ReactionResource::d_yield_nuclides.
|
virtual |
direct yields
References ReactionResource::d_direct_yields.
Referenced by Origen::populateReactionResource_AmpxLibrary().
|
virtual |
set new direct yields
References ReactionResource::d_direct_yields.
|
virtual |
remove direct yields
References ReactionResource::d_direct_yields.
void import_fission_yields | ( | const YieldResource & | yr | ) |
References ReactionResource::d_yield_nuclides, YieldParent::data_at(), YieldData::energy(), YieldResource::fissionable_at(), YieldParent::get_fp_ids(), YieldParent::id(), YieldParent::num_energies(), YieldResource::num_fissionables(), YieldData::num_yields(), YieldData::yield_at(), Origen::YIELD_DISTRIBUTED, and Origen::YIELD_ISOMERIC.
const Vec_Flt & storage_bounds | ( | ) | const |
return a const reference to storage_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of ReactionResource goes out of scope.
Referenced by TransitionMatrixUpdater::num_energy_groups(), and TransitionMatrixUpdater::storage_bounds().
Vec_Flt::value_type storage_bounds_at | ( | size_t | i | ) | const |
get element of storage_bounds vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
SCP_Vec_Flt scp_storage_bounds | ( | ) | const |
return a shared (read-only) storage_bounds pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Vec_Flt::value_type const * storage_bounds_array | ( | ) | const |
return view of storage_bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
size_t storage_bounds_size | ( | ) | const |
return size of storage_bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by ReactionResource::canonicalize_library_flux(), and ReactionResource::canonicalize_storage_flux().
bool has_storage_bounds | ( | ) | const |
return whether the class has a non-null storage_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by ReactionResource::canonicalize_library_bounds(), and ReactionResource::canonicalize_storage_flux().
void set_storage_bounds | ( | const Vec_Flt & | storage_bounds | ) |
set the storage_bounds (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by ReactionResource::ReactionResource().
void get_storage_bounds | ( | Vec_Flt & | storage_bounds | ) | const |
populate an existing Vec_Flt with a copy of storage_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void manageptr_storage_bounds | ( | Vec_Flt *& | storage_bounds | ) |
set the storage_bounds (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void getptr_storage_bounds | ( | Vec_Flt const *& | storage_bounds | ) | const |
retrieve pointer (read-only) to storage_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_storage_bounds_array | ( | size_t | , |
float * | |||
) | const |
get array of storage_bounds vector (Fortran)
void set_storage_bounds_array | ( | size_t | , |
float * | |||
) |
set storage_bounds vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
const Vec_Flt & storage_flux | ( | ) | const |
return a const reference to storage_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of ReactionResource goes out of scope.
Referenced by ReactionResource::canonicalize_library_flux().
Vec_Flt::value_type storage_flux_at | ( | size_t | i | ) | const |
get element of storage_flux vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
SCP_Vec_Flt scp_storage_flux | ( | ) | const |
return a shared (read-only) storage_flux pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Vec_Flt::value_type const * storage_flux_array | ( | ) | const |
return view of storage_flux vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
size_t storage_flux_size | ( | ) | const |
return size of storage_flux vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
bool has_storage_flux | ( | ) | const |
return whether the class has a non-null storage_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_storage_flux | ( | Vec_Flt & | storage_flux | ) | const |
populate an existing Vec_Flt with a copy of storage_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void getptr_storage_flux | ( | Vec_Flt const *& | storage_flux | ) | const |
retrieve pointer (read-only) to storage_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_storage_flux_array | ( | size_t | , |
float * | |||
) | const |
get array of storage_flux vector (Fortran)
const Vec_Flt & library_bounds | ( | ) | const |
return a const reference to library_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of ReactionResource goes out of scope.
Vec_Flt::value_type library_bounds_at | ( | size_t | i | ) | const |
get element of library_bounds vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
SCP_Vec_Flt scp_library_bounds | ( | ) | const |
return a shared (read-only) library_bounds pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Vec_Flt::value_type const * library_bounds_array | ( | ) | const |
return view of library_bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
size_t library_bounds_size | ( | ) | const |
return size of library_bounds vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
Referenced by ReactionResource::canonicalize_library_bounds(), and ReactionResource::canonicalize_library_flux().
bool has_library_bounds | ( | ) | const |
return whether the class has a non-null library_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by ReactionResource::canonicalize_library_flux().
void set_library_bounds | ( | const Vec_Flt & | library_bounds | ) |
set the library_bounds (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by Origen::populateReactionResource_AmpxLibrary().
void get_library_bounds | ( | Vec_Flt & | library_bounds | ) | const |
populate an existing Vec_Flt with a copy of library_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void manageptr_library_bounds | ( | Vec_Flt *& | library_bounds | ) |
set the library_bounds (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void getptr_library_bounds | ( | Vec_Flt const *& | library_bounds | ) | const |
retrieve pointer (read-only) to library_bounds
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_library_bounds_array | ( | size_t | , |
float * | |||
) | const |
get array of library_bounds vector (Fortran)
void set_library_bounds_array | ( | size_t | , |
float * | |||
) |
set library_bounds vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
const Vec_Flt & library_flux | ( | ) | const |
return a const reference to library_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Invalid if this instance of ReactionResource goes out of scope.
Vec_Flt::value_type library_flux_at | ( | size_t | i | ) | const |
get element of library_flux vector by index
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
SCP_Vec_Flt scp_library_flux | ( | ) | const |
return a shared (read-only) library_flux pointer
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Vec_Flt::value_type const * library_flux_array | ( | ) | const |
return view of library_flux vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
size_t library_flux_size | ( | ) | const |
return size of library_flux vector
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
bool has_library_flux | ( | ) | const |
return whether the class has a non-null library_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void set_library_flux | ( | const Vec_Flt & | library_flux | ) |
set the library_flux (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by Origen::populateReactionResource_AmpxLibrary().
void get_library_flux | ( | Vec_Flt & | library_flux | ) | const |
populate an existing Vec_Flt with a copy of library_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void manageptr_library_flux | ( | Vec_Flt *& | library_flux | ) |
set the library_flux (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void getptr_library_flux | ( | Vec_Flt const *& | library_flux | ) | const |
retrieve pointer (read-only) to library_flux
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
void get_library_flux_array | ( | size_t | , |
float * | |||
) | const |
get array of library_flux vector (Fortran)
void set_library_flux_array | ( | size_t | , |
float * | |||
) |
set library_flux vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
void map_xs | ( | Vec_Flt * | xs | ) | const |
void map_flux | ( | Vec_Flt * | flux | ) | const |
bool using_data_mapper | ( | ) | const |
References ReactionResource::d_using_data_mapper.
|
staticprotected |
Referenced by ReactionResource::ReactionResource().
|
staticprotected |
References ReactionResource::max_isomeric_state().
Referenced by ReactionResource::ReactionResource().
|
staticprotected |
Referenced by ReactionResource::ReactionResource().
|
protected |
set the storage_flux (copy operation)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
Referenced by ReactionResource::canonicalize_library_flux().
|
protected |
set the storage_flux (ownership transfer)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_OBJECT().
|
protected |
set storage_flux vector from array (Fortran)
Auto-generated from macro #ORIGEN_ACCESSORS_SP_VECTOR().
|
protected |
|
protected |
|
protected |
|
protected |
References ReactionResource::d_data_mapper, ReactionResource::d_library_bounds, ReactionResource::d_library_flux, ReactionResource::d_storage_bounds, ReactionResource::d_storage_flux, ReactionResource::d_using_data_mapper, ReactionResource::has_library_bounds(), ReactionResource::library_bounds_size(), ReactionResource::set_storage_flux(), ReactionResource::storage_bounds_size(), and ReactionResource::storage_flux().
Member Data Documentation
|
protected |
|
protected |
|
protected |
|
protected |
|
protected |
Referenced by ReactionResource::canonicalize_library_flux().
|
protected |
|
protected |
|
protected |
|
protected |
|
protected |
|
inherited |
STL member.
|
inherited |
STL member.
The documentation for this class was generated from the following files:
- Core/re/ReactionResource.h
- Core/re/ReactionResource.cpp